Sunday, March 26, 2006
Saturday, March 25, 2006
Comp 471: Asg 4: Smoke

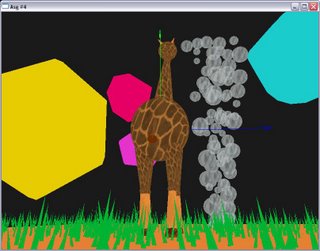
Description: In my Comp471 Asg4, they ask to either do motion blur on the wagging tail (haha), OR to create smoke coming out of a bush fire of some sort. After spending the morning learning about blur techniques, I found out how (explained bellow). However, the thought of making smoke seemed like a better choice considering the creation fun factor. Anyway, I thought the best way to do smoke for this assignment was with spheres! (looks cartoonish and easy to implement, since its a primitive type). Re-Anway, read the details.
Details:
- Everything is dynamically created and animated (which means I can add, remove, change, move differently, etc);
- For now, limited amount of smoke (the longer lasting smoke, the less fps);
- The texture is nothing special (but that wasn't the point, neither was making a nice giraffe..);
TODO:
- Try a different style of smoke (much smaller spheres, but more of them);

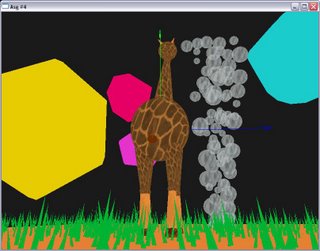
Description: In my Comp471 Asg4, they ask to either do motion blur on the wagging tail (haha), OR to create smoke coming out of a bush fire of some sort. After spending the morning learning about blur techniques, I found out how (explained bellow). However, the thought of making smoke seemed like a better choice considering the creation fun factor. Anyway, I thought the best way to do smoke for this assignment was with spheres! (looks cartoonish and easy to implement, since its a primitive type). Re-Anway, read the details.
Details:
- Everything is dynamically created and animated (which means I can add, remove, change, move differently, etc);
- For now, limited amount of smoke (the longer lasting smoke, the less fps);
- The texture is nothing special (but that wasn't the point, neither was making a nice giraffe..);
TODO:
- Try a different style of smoke (much smaller spheres, but more of them);
Friday, March 24, 2006
My College Game Project:
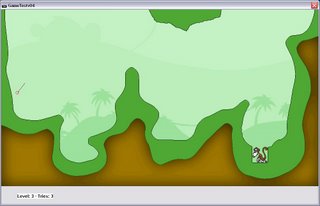
-This is game project I designed and implemented in College;
-The drawings of the maps were done by a friend;
- The background and monkey were "stolen" from the net;
Description:
- You adjust angle and velocity of your cannon;
- You shoot a ball that bounces around;
- The ball must land in the monkey's whole;
- If you miss, you have 2 other tries. And you start from where it landed;
- There are 5 levels, ranging from eassy to difficult;
Details:
- Collision detection is done with my own algorithm: 12 point collision detection;
+ It checks for color differences in the maps on 12 points around the ball.
- Physics are implemeted (except there is no rolling up-hill)
+ Ball composition can be easily altered (rubber, metal, etc)
Wanted to do:
- Add levels in desert and in winter (ran out of time);
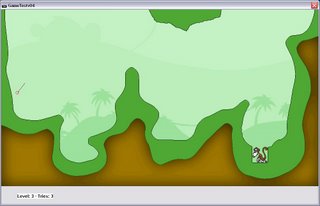
-This is game project I designed and implemented in College;
-The drawings of the maps were done by a friend;
- The background and monkey were "stolen" from the net;
Description:
- You adjust angle and velocity of your cannon;
- You shoot a ball that bounces around;
- The ball must land in the monkey's whole;
- If you miss, you have 2 other tries. And you start from where it landed;
- There are 5 levels, ranging from eassy to difficult;
Details:
- Collision detection is done with my own algorithm: 12 point collision detection;
+ It checks for color differences in the maps on 12 points around the ball.
- Physics are implemeted (except there is no rolling up-hill)
+ Ball composition can be easily altered (rubber, metal, etc)
Wanted to do:
- Add levels in desert and in winter (ran out of time);
My AI Project: Part 1
These are copies of an AI mind I've coded.
- There is the main BOT (spheres), then there is their direction/speed vector (short line). And finaly, the IR (infra-red) radar (long line).
It all started out when me and a friend decided to create an AI controlled robot in real life. So I started on my part on the PC, while my friend is working on the electronics.
So when I was creating these bots, I was keeping in mind the fact I'd have to use that AI on a real robot.
So for example, collision detection in real life will be done with an IR transmitter and receiver. So...in my digital version, I simulate a rotating IR transmitter and receiver. (transmitter is the long line, receiver is the collision detection algorithm)
In short: They go about randomly, and when they encounter a wall (red) they break then steer in order to avoid it. They will do this forever.
Details: The IR spins around the bot, checking each angle for a wall. When a wall is "touched" by the radar, the bot brakes. Once it is going slow enough, it steers away from the wall and pics a new direction. -- When not "seing" any wall, the bot randomly chooses a new direction to explore.
-- The walls are detected using polygon-line collision detection. So walls can be placed anywhere and still be detected.
Problems:
- After lots of time, they start to get stuck, because the IR is not spinning fast enough.
Must think about this because in real life, we can't have the IR spin too fast.
Next Part:
- Make the IR "see" other bots as well as walls;
- Create a bigger and tougher to navigate level (map);
- Add battery life, and a charging center, where the bot will go to recharge when low on battery;
- Design and implement a better AI:
+ Bots will rememeber walls once they encounter them;
+ Bots will be able to go from A to B watching for new walls and the walls in memory;
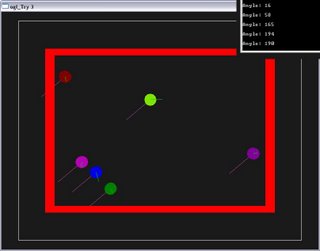
- There is the main BOT (spheres), then there is their direction/speed vector (short line). And finaly, the IR (infra-red) radar (long line).
It all started out when me and a friend decided to create an AI controlled robot in real life. So I started on my part on the PC, while my friend is working on the electronics.
So when I was creating these bots, I was keeping in mind the fact I'd have to use that AI on a real robot.
So for example, collision detection in real life will be done with an IR transmitter and receiver. So...in my digital version, I simulate a rotating IR transmitter and receiver. (transmitter is the long line, receiver is the collision detection algorithm)
In short: They go about randomly, and when they encounter a wall (red) they break then steer in order to avoid it. They will do this forever.
Details: The IR spins around the bot, checking each angle for a wall. When a wall is "touched" by the radar, the bot brakes. Once it is going slow enough, it steers away from the wall and pics a new direction. -- When not "seing" any wall, the bot randomly chooses a new direction to explore.
-- The walls are detected using polygon-line collision detection. So walls can be placed anywhere and still be detected.
Problems:
- After lots of time, they start to get stuck, because the IR is not spinning fast enough.
Must think about this because in real life, we can't have the IR spin too fast.
Next Part:
- Make the IR "see" other bots as well as walls;
- Create a bigger and tougher to navigate level (map);
- Add battery life, and a charging center, where the bot will go to recharge when low on battery;
- Design and implement a better AI:
+ Bots will rememeber walls once they encounter them;
+ Bots will be able to go from A to B watching for new walls and the walls in memory;
Comp 471: Asg 4


Description:
- Lighted, textured and with FX.
Details:
- Added three lights:
+ A white head light on the giraffe;
+ A red user light (comes out of 3d-person camera);
+ A blue spinning light (around the giraffe facing down);
ToDo:
- Texture the giraffe (Different textures for different body parts);
- Create a mettalic shine and rusty appearances (Be able to switch between the two);
- Add motion blur as an FX;


Description:
- Lighted, textured and with FX.
Details:
- Added three lights:
+ A white head light on the giraffe;
+ A red user light (comes out of 3d-person camera);
+ A blue spinning light (around the giraffe facing down);
ToDo:
- Texture the giraffe (Different textures for different body parts);
- Create a mettalic shine and rusty appearances (Be able to switch between the two);
- Add motion blur as an FX;
Comp 471: Asg 3

Description:
Giraffe in an environment.
Details:
- Grass is dynamically generated;
- Simulation of gentle breeze on grass;
- Giraffe is made of primitives;
- Can move head and neck;
- It can walk forwards and backwards;
- It's belly scales horizontaly to simulate breathing;
- It wags its tail on command;
- Primitives are just for view relativity;

Description:
Giraffe in an environment.
Details:
- Grass is dynamically generated;
- Simulation of gentle breeze on grass;
- Giraffe is made of primitives;
- Can move head and neck;
- It can walk forwards and backwards;
- It's belly scales horizontaly to simulate breathing;
- It wags its tail on command;
- Primitives are just for view relativity;

OpenGL: Plane out of Triangles
pS: not my algorithm.
void drawPlane(int w, int h)
{
int i, j;
float dw = 1.0 / w;
float dh = 1.0 / h;
glNormal3f(0.0, 0.0, 1.0);
for (j = 0; j < h; ++j) {
glBegin(GL_TRIANGLE_STRIP);
for (i = 0; i <= w; ++i) {
glVertex2f(dw * i, dh * (j + 1));
glVertex2f(dw * i, dh * j);
}
glEnd();
}
}